Update: Fixed non-working code. Thanks to Dmitriy for pointing out the issue.
Preface
In this tutorial, we will look at 2 ways to handle button press:
- Consecutive
- Parallel.
Let’s, begin.
Sequential way of processing clicks.
Create a handleInput() function, inside the main.c of your project. And rewrite the following code there.
void handleInput(){
VDP_clearText(1,1,5);
u16 value = JOY_readJoypad(JOY_1);
if(value & BUTTON_LEFT) {
VDP_drawText("Left",1,1);
}
if(value & BUTTON_RIGHT) {
VDP_drawText("Right",1,1);
}
}
Let’s consider the new commands:
VDP_clearText(1,1,5);
This command removes tiles from the specified area. Its syntax is as follows:
VDP_clearText(x_tile, y_tile, length);
- x_tile – indicates the tile on the x-axis
- y_tile – sets the tile on the y axis
- length – specifies the length of the area to be removed.
It turns out that this command
VDP_clearText(1,1,5);
We removed tiles in area 1.1 to 1.5 (inclusive).
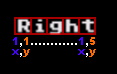
u16 value = JOY_readJoypad(JOY_1);
- Type u16, can be represented as unsigned 16, which means an unsigned 16-bit number.
- The function JOY_readJoypad,specifies the joystick (JOY_1) from which we will read the information, and returns information about the buttons pressed.
if(value & BUTTON_LEFT) {
VDP_drawText("Left",1,1);
}
Here, using a bit mask(BUTTON_LEFT), we checked whether the button to the leftwas pressed. If pressed, display the text on the screen.
if(value & BUTTON_RIGHT) {
VDP_drawText("Right",1,1);
}
Here, the same thing, only for the button to the right.
A list of bitmap masks is available at installation_path_SGDK/doc/html/joy_8h.html
The function is ready, it remains to call it inside the main.
int main()
{
while(1)
{
handleInput();
SYS_doVBlankProcess();
}
return (0);
}
Now, compile,run, and click left, right. When you click on the button, the text on the screen should appear.
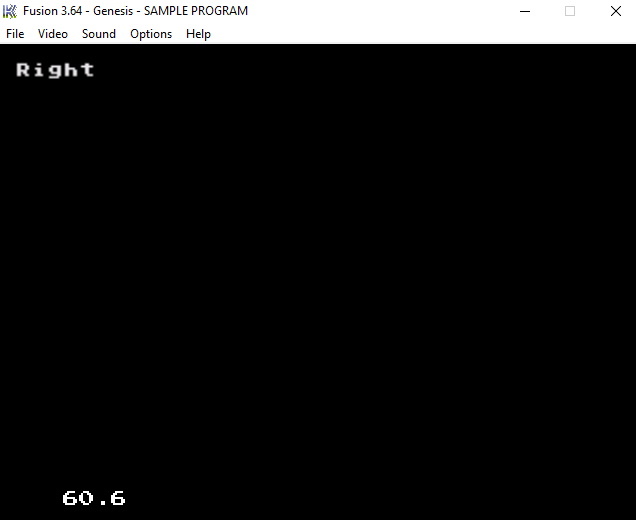
Now, let’s analyze the second way of processing clicks.
Parallel way of processing clicks.
This method, unlike the previous one, does not require a constant call of the function. Because, it is based onevent handlers (Event Handler). The algorithm here is as follows, create a callback function, pass it to another function, and that’s it.
A callback function is a function that another function takes as an argument.
Create a new function inside the main.c of your project.
void handleInputSecond( u16 joy, u16 changed, u16 state) {
VDP_clearText(1,1,5);
if(joy == JOY_1) {
if(state & BUTTON_LEFT) {
VDP_drawText("Left",1,1);
}
if(state & BUTTON_RIGHT) {
VDP_drawText("Right",1,1);
}
}
}
Let’s analyze this code:
void handleInputSecond( u16 joy, u16 changed, u16 state)
This function takes 3 arguments:
- joy – the number that those that quench the joystick from which the key was pressed. (JOY_1, JOY_2)
- changed – if the key pressed has changed, returns 1,otherwise 0.
- state – sets the key pressed.
The rest of the code, we parsed above. Now, let’s create an event handler inside main.
int main()
{
JOY_init();
JOY_setEventHandler(&handleInputSecond);
while(1)
{
SYS_doVBlankProcess();
}
return (0);
}
Let’s analyze the code.
JOY_init();
Makes the initial adjustment of the joystick (without this command, this method will not work).
JOY_setEventHandler(&handleInputSecond);
Here we passed our event handler(callBack function).
That’s it, done. The second method, in terms of functionality, is no different from the first. So, choose what you want.
Final result.
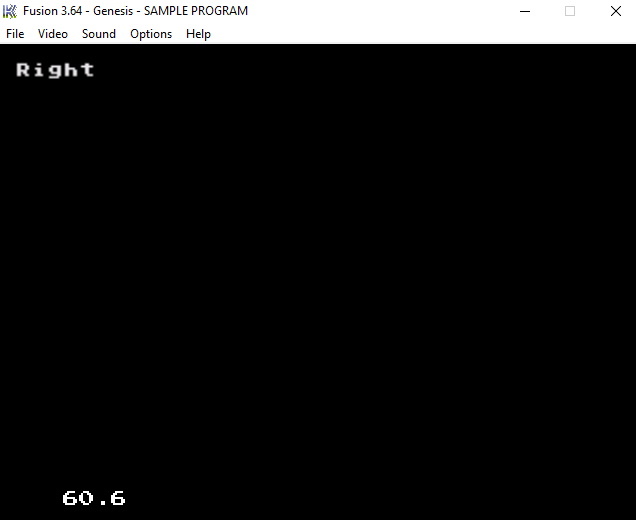